Mostly Used Commands In Github
Distributed version control(Github) vs
centralized version control(SourceSafe): Centralized version control means that the version
history is stored in a central server. By contrast, Github is
a type of version control where the complete codebase — including
its full version history — is mirrored on every developer's
computer. It's abbreviated DVCS.
In DVCS, files can be stored in 4 areas:
Worktree( or work
directory) - Index/Stage - Repository - Remote.
The status of a file can be:
modified, staged, committed.
Attention: some commands will become deprecated or no longer
supported after a period of time. Please always refer to github
latest manual.
Configuration
There are three levels of config: system( all users), global( all repositories of current user), local( the current repository)-
Global Config
// show the current git config git config - - list // open and edit .gitconfig file git config — -global -e git config — - global user.name “Your Name” git config — - global user.email "email@example.com" git config — -global core.editor “code - - wait “ // set vscode as source editor git config - -global diff.tool vscode // The following setup should leave you with CRLF endings in Windows checkouts, but LF endings on macOS and Linux systems and in the repository. // for macOS git config —global core.autocrlf input // for windows git config —global core.autocrlf true
-
Local (or project specific) config
Project configs are available for the current project and stored in .git/config in the project's directory. Create a project specified config, you have to execute this under the project's directory:
git config user.name "Your name"
create a local repository
// in the current directory git init git add REAMDE.md // create or replace a branch git branch -M main // if error: refname refs/heads/master not found // You are currently in detached head state. // You must checkout a new branch to associate it with the current commit: // create a new branch if it does not exist, then checkout it git checkout -b newbranch // create a new branch if it does not exist; if it exists, reset it. git checkout -B newbranch // create a local branch and track the remote branch with the same name // we can omit <branch> // git checkout -b Alice/#newFeature origin/Alice/#newFeature $ git checkout -b <branch> <remote>/<branch> // clone a remote repository : method 1 git clone url // clone a remote repository method 2 git remote add [repo-short-name] url // e.g. git remote add origin https://github.com/your-user-name/your-project-name.git // change up-stream repo of local project git remote set-url [repo-short-name] url // e.g git remote set-url origin https://github.com/your-user-name/your-project-name.git // show remote repository list git remote // change remote name git remote rename [old-upstream-name] [new-up-stream-name]
.gitignore
A . gitignore file is a plain text file where each line contains a pattern for files/directories to ignore. Generally, this is placed in the root folder of the repository. However, you can put it in any folder in the repository and you can also have multiple . gitignore files.
// patterns in .gitignore logs/ main.log *.log # dependencies /node_modules coverage/ config/ // website https://github.com/github/gitignore includes .gitignore samples for different languages.
add or delete files
// add files to stage area git add [file1] [file2] ... // add an folder(including sub-folder) git add [folder1] // add all files in the current directory to stage area git add . // If a file or folder has been tracked, add the pattern to .gitignore is not enough. // We should also delete them from staging area(not from worktree) // -r: recursive git rm — - cached -r foldername git rm - - cached filename // delete a file from worktree, and add the delete operation to stage area git rm [file1] [file2] ... // rename a file, add the operation to stage area git mv oldname newname
commit - submit the changes
// add the files that have changed from last commit git commit -a git commit -a [file1] // submit changes from stage area to repository git commit -m [message] // submit the specified file in stage area to repository git commit file1 [file2] ... -m [message] // shorthand git commit -a + git commit -m git commit -am [message] // submit and show diff git commit -v // replace the previous commit by the new commit // if files do not change, amend only the message git commit -amend -m [message]
show changes
// list all fils at the staging area git ls-files git status // show the changes in worktree and staging area git status -s // show difference between staging area files and worktree file git diff // show difference between worktree and last commit of current branch git diff HEAD // what happened at staging area, including staged but not committed files git diff - - cached // --staged is a synonym of --cached git diff - - staged // show difference between 2 commits // e.g. abbreviated commit id : 5efb3c8 // e.g. complete commit id: a5a4df9c77b8d7d7b0a693d113a7fb3e6eaa11c6 git diff commit1Id commit2Id git diff HEAD~2 HEAD filename // show difference between 2 branches git diff [master-branch] [other-branch] // show changes in a specified commit git show [commit-id] // show changes in a file of a commit git show [commit-id]:[file-name] // show commit log of current branch, including "git reset" git reflog
branch
// list all local branches git branch // list all remote branches git branch -r // list all local and remote branches git branch -a // create a branch and switch to it git switch -c [new-branch-name] // This is a convenient shortcut for: git branch [new-branch] git switch [new-branch] // create or replace a branch and switch to it git switch -C new-branch-name // This is a convenient shortcut for: git branch -f [new-branch] git switch [new-branch] // create a branch and track a remote branch git switch -C [local-new-branch] origin/[remote-branch-name] // set tracking a remote branch git branch --set-upstream-to=origin/[remote-branch] [local-branch] e.g. git branch --set-upstream-to=origin/new-feature new-feature // a remote branch can be created by push if not exist git push -u( - -up-stream) origin [local-new-branch] // merge a specified branch to current branch // merge is non-destructive operation. The existing branches kept all their commit history. git merge [will-be-merged-branch] git branch - - merged git branch - - no-merged // flatten and simplify a commit history tree. put all changes from merged branch into stage area of main branch git merge - - squash [will-be-merged-branch] // git rebase moves the entire feature branch to begin at the top of another branch // it simplifies but rewrites the project commit history git rebase [another-branch] // if a branch has multiple commits, it is possible to pick up a specified commit git cherry-pick [specific-commit-id-from-unmerged-branch] // delete a branch git branch -d [branch-name] // delete a branch, even if not merged git branch -D [branch-name] // delete a remote branch git push origin - - delete [remote-branch-name] git push -d origin [remote-branch-name] // e.g. delete remote/origin/test git push origin - - delete test // before switch to another branch, save but not commit changes git stash push -m "change description" // switch back to old branch and show stash git switch [branch-name] git stash list git stash show 1 git stash apply 1 git stash drop 1 git stash clear // shorthand of git stash apply + git stash drop git stash popgit merge
Reference: https://www.atlassian.com/git/tutorials/merging-vs-rebasing
tag
// list all tags git tag // add tag to last commit git tag [tag-name] // add tag to a specified commit git tag [tag-name] [commit-id] // show tag information git show [tag-name] // push a specified tag to remote git push origin [tag-name] // delete a local tag git tag -d [tag-name] // delete a remote tag git push origin - - delete [remote-tag-name]
show history of commit
git log git log - - oneline git log - -oneline - -all - -graph // display added, modified, and updated location in a specified file git log - - patch git log -p git log - - patch [file-name] // list history in reverse chronological order git log - - oneline - - reverse // show detailed changes in a specified commit git show [commit-id] // go back one step from last commit git show HEAD~1 filename // show latest commit git show HEAD // show all files in this commit git ls-tree HEAD~1 // filter the log by date git log --after="yy-mm-dd" // e.g git log --after="2017-01-31" // find the author names of a file git blame -e [file-name] // only show the first 3 lines of commit history git blame -e L 1,3 [file-name]
synchronize local and remote repositories
// step1: get changes in remote repository git fetch [remote-repository-name] // step2: update a specific file using the file in remote repo git checkout [remote-repository-name] [local-path/filename] // example git checkout origin/master README.md // show all remotes git remote -v // output in terminal, for example : // heroku https://git.heroku.com/remote-repository-name.git (fetch) // origin https://github.com/your-username-in-github/remote-repository-name2.git (fetch) // show a specific repository detailed information git remote show [remote-repository] // enter the following command in terminal : // git remote show https://github.com/your-username-in-github/remote-repository-name2.git // output in terminal : // * remote https://github.com/your-username-in-github/remote-repository-name2.git // HEAD branch: master // add a new remote repository git remote add [repo-name] [url] // remove a remote repo from local git remote remove [repo-name] // e.g git remote remove origin // integrate remote change into local branch git pull [remote] [branch] // upload local changes to remote git push [remote] [branch] // e.g. git push origin master // push the local branch to an remote branch with a different name in remote repo git push origin [local-branch-name]:[remote-branch-name] // merge a remote branch to current local branch-name git fetch git merge [remote-repo-local-name]/[remote-branch-name] // delete a branch in remote repo git push origin :[remote-branch-name] // update remote with local change even if there are conflicts git push [remote] --force // push all branches to remote git push [remote] - - all // change the remote repo's local name git remote rename [old-name] [new-name] e.g. git remote rename origin org // keep local branches in sync with remote branches. If a branch has been deleted from remote repo, after executing this command, // the branch will be deleted from your local repo. git remote prune [remote] // e.g. git remote prune origin
revert changes
// use case: changes exist in worktree, not in stage // restore a specified file from stage to worktree, give up the changes in worktree git checkout [filename] git restore [filename] / use case: changes exist in worktree, not in stage // restore a file to worktree from a specified commit git checkout [commit-id] [file-name] // use case: changes exist in worktree and have been added to stage // we need 2 steps to revert it git reset HEAD [file-name] git checkout [file-name] // restore all files into worktree from last commit. It means giving up the changes from last commit. git checkout . // reset the current HEAD to a specified state // reset file in stage area with content from last commit, not reset file in worktree git reset [file-name] // reset stage and worktree at the same time git reset - - hard [file-name] // reset the current HEAD to a specified commit, reset stage area, not reset worktree git reset [commit-id] git reset - - soft [commit-id] git reset - - soft HEAD~1 // reset stage and worktree to a specified commit git reset - -hard [commit-id] // reset current HEAD, not change the content in stage and worktree git reset - - keep [commit-id] // declare and automatically execute a new commit to undo all changes in the specified commit git revert [commit-id] // declare a new revert commit but not automatically execute git revert - -no-commit [commit-id] git revert — - no-commit HEAD~3 // revert multiple commits git revert HEAD~3..HEAD
pull request
Pull requests help you collaborate on code with other people. You
forked a repo from other's repositories. After adding new features
to the repo, you think of merging them into the original repo.
Then you should create a pull request. The owner of the original
repo can see the pull request and decide to merge it or not.

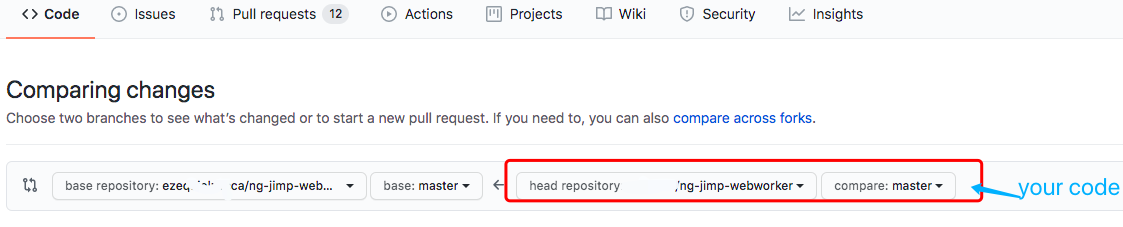